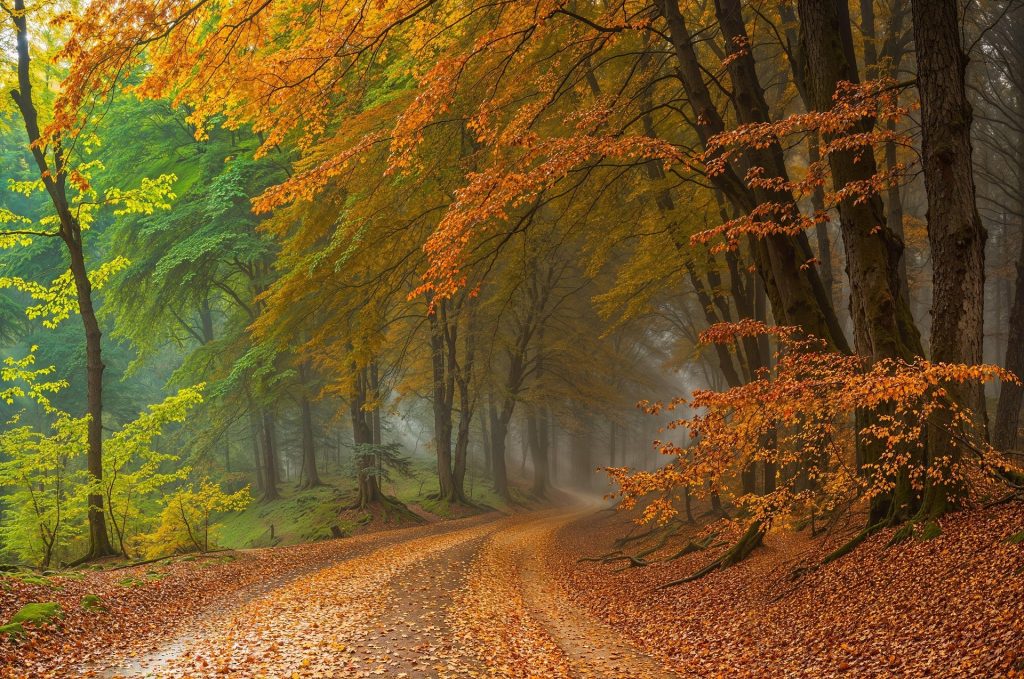
Understanding Algorithms and Data Structures for Beginners
When stepping into the world of programming, two terms often come up: algorithms and data structures. While they might sound complex at first, they are fundamental concepts that every aspiring programmer needs to grasp. This article aims to break them down into digestible portions for beginners.
What are Algorithms?
An algorithm is essentially a step-by-step procedure or formula for solving a problem. Think of it like a recipe: just as a recipe outlines the steps for preparing a dish, an algorithm outlines a series of steps to achieve a specific goal, such as sorting a list of numbers or searching for a particular item in a dataset.
Algorithms can vary in complexity. Some are straightforward, like finding the maximum number in a list. Others can be more intricate, involving multiple steps and decision points. Regardless of complexity, the key characteristics of a good algorithm include:
- Clear and unambiguous: Each step should be well-defined.
- Well-defined inputs and outputs: It should specify what data it takes in and what results it produces.
- Finiteness: An algorithm must always end after a finite number of steps.
- Effectiveness: The steps should be basic enough that they can be performed, in principle, by a human using paper and pencil.
Types of Algorithms
Algorithms can be categorized in various ways. Here are a few common types:
-
Sorting Algorithms: These arrange data in a particular order, like numerical or lexicographic. Examples include Bubble Sort, Merge Sort, and Quick Sort.
-
Search Algorithms: These help find specific data from a data structure. For instance, Linear Search checks each item one by one, while Binary Search divides the dataset in half to find the target more efficiently.
-
Recursive Algorithms: These call themselves with modified arguments to solve a smaller instance of the problem, useful for tasks like calculating factorials or traversing tree structures.
- Dynamic Programming: This technique breaks down problems into smaller subproblems and stores the results to avoid redundant calculations, which is beneficial for optimization problems.
What are Data Structures?
Data structures are ways of organizing and storing data so that it can be accessed and modified efficiently. Choosing the right data structure can significantly impact the performance of your algorithms.
Some commonly used data structures include:
-
Arrays: A collection of elements identified by index numbers. Arrays are fixed in size, making them straightforward but limiting in flexibility.
-
Linked Lists: A sequence of elements, where each element points to the next. This allows for dynamic memory allocation, making it easier to add or remove elements compared to arrays.
-
Stacks: A collection that follows the Last In, First Out (LIFO) principle. Think of it like a stack of plates: you can only add or remove the top plate.
-
Queues: A collection that follows the First In, First Out (FIFO) principle. Like a line of people waiting for a bus, the first to arrive is the first to leave.
-
Hash Tables: These associate keys with values for quick data retrieval. They can provide average-case time complexity for search and insert operations.
-
Trees: A hierarchical structure that consists of nodes, starting from a root node and branching out. Binary trees, where each node has at most two children, are a common example.
- Graphs: Collections of nodes (vertices) and edges connecting them. Graphs are used to represent a variety of scenarios, such as social networks or GPS navigation.
The Relationship Between Algorithms and Data Structures
The relationship between algorithms and data structures is crucial to effective programming. The choice of data structure can significantly influence the performance of an algorithm. For instance, searching through a sorted array can be done quickly using binary search, but if the data were in a linked list, the search would be much slower.
Understanding both concepts allows developers to write efficient and optimized code. It enables them to pick the right tools for the job, ensuring that programs run smoothly and effectively.
In summary, mastering algorithms and data structures is essential for any programmer. They influence how problems are solved and help create efficient, high-performance applications. As you embark on your coding journey, becoming familiar with these concepts will empower you to tackle more complex challenges with confidence and skill. Whether you are building simple applications or tackling complex systems, the foundation of algorithms and data structures will support your growth as a developer.